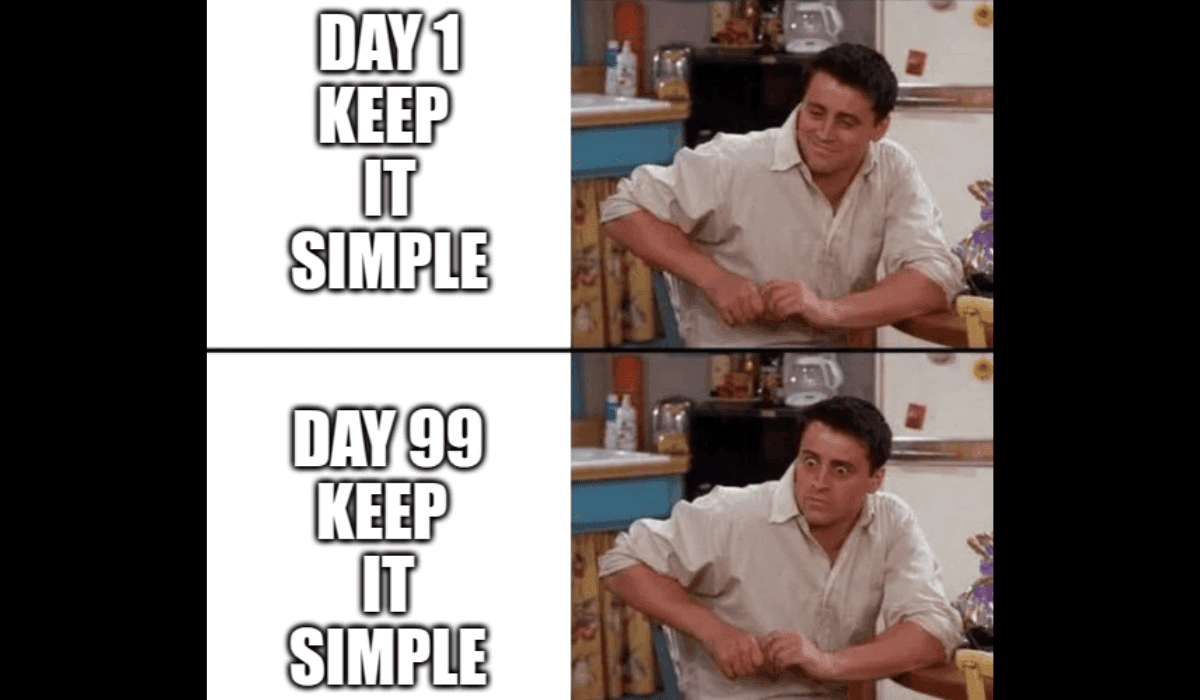
Why Simplicity Is the Hardest Thing to Build in Programming
KISS is not just a nice word — it stands for "Keep It Simple, Stupid." It’s a design principle that reminds us to avoid unnecessary complexity. But in real-world programming, simplicity is often the hardest thing to achieve. It sounds easy in theory, but when deadlines, team discussions, feature requests, and legacy code come into play, simplicity becomes a real challenge.
Let’s talk about why that happens.
Simplicity Takes More Time, Not Less
Many people think simple code means fast or easy code. It’s the opposite.
Simple code takes time to think through. You have to:
- Understand all the dimensions and angle of requirement.
- Know the future requirement.
- Strip away what's not needed.
- Pick the right structure — not the fastest one to write.
- Train and enlighte the team.
Most deadlines don’t give you this luxury. So, developers often go with the solution that works now, not the one that’s simple long term.
To be more accurate, simplicity takes more time initially, but saves time later. The reason being simple, the simple&neat code is easier to read, understand, maintain, extend, and migrate.
In case of complex code, it leads to slow death of the project. This might sound dramatic, but it’s reality. Complex code is like a tangled web. The more you add, the harder it is to change anything without breaking something else.
It’s like trying to untangle a ball of yarn. You pull one thread, and suddenly you have a mess on your hands. For more details, check out how-did-kiss-change-the-way-i-code.
Another aspect is developers mantality, if from start developer starting adopting KISS then eventually it become second nature to him, and this can boost the time.
Complexity Is a Side Effect of Rushing
Imagine building a login system. You start with just username and password. Then the team asks:
- Can we support social logins too?
- How about multi-factor authentication?
- Let’s log all failed login attempts for security.
Suddenly, what started simple becomes a jungle. You keep adding code instead of thinking:
"Is there a better way to structure this from the start?"
The pressure to "just get it working" leads to systems that are harder to read, test, and maintain.
More Code Feels Like More Work
Developers often feel proud of writing lots of code. It's visible effort.
But simple code means:
- Fewer lines.
- Fewer classes.
- Fewer conditions.
And that doesn’t always feel like you're doing enough — especially in a team where output is often measured by how much you’ve shipped.
But experienced developers know:
The best code is the one you don’t have to write.
But you might not agree with this point, cause not everywhere this apply unless your manager ask why it taken so much time writting just 20 lines of code?.
It’s Hard to Say “No”
Most complexity comes from trying to welcome everyone:
- PMs want more features.
- Designers want more options.
- QA wants more edge cases covered.
Simplicity requires saying “no” or “not now” — and that’s not easy in a team. But without strong boundaries, complexity grows silently. for more details, check out how-to-say-no.
Simplicity Requires Understanding the Problem, Not Just the Tools
Many developers jump to solutions before they truly understand the problem.
You might say:
“Let’s use Redis for caching!”
But… do you need caching? Or is the slowness from a bad SQL query?
Tools add layers. Simplicity comes when you fully understand what needs solving, and then do the least required to fix it.
Simplicity is way of thinking, crafting, enjoying. But we assume that if we followed KISS then will be easy to add and extend but KISS not just apply at code level but at all places of software cycle, starting from way of seeing requirements and problem from different perspective, angle, dimension.
Simple Code Is Often Hard to Write, but Easy to Read
Good code reads like a story:
if user.is_logged_in() and user.has_access():
show_dashboard()
You instantly know what it’s doing.
Now compare with:
if session.get("uid") and permissions[user_id]["level"] > 4:
do_stuff()
Same logic. But the second version makes you pause. Clean, simple code may take longer to write, but it saves hours for the next person — even if that’s future you.
Great Products Hide Complexity
Think about:
- Google Search: One box, one button. Billions of dollars of engineering behind it.
- Stripe API: One line of code to take payments. Years of thought went into making it feel “easy.”
- Unix Philosophy: Each tool does one thing well. That's why small shell tools like
grep
,cat
, andawk
still exist after 40+ years.
These examples show: simplicity is not a lack of depth — it's deep mastery, expressed with clarity.
Conclusion
Simplicity in programming is not about being lazy. It’s about being smart. It’s about making things easier to understand, use, and maintain. It’s hard because it requires experience, empathy, and restraint.
If you’re a beginner, don’t worry if your code isn’t simple yet. Simplicity is not where you start — it’s where you arrive, after learning what doesn’t work.
Next time you're about to ship something, ask yourself:
“Can I make this simpler?”
That one question could save you — or your team — weeks down the road.
And i highly recommend to read
- The Art of Readable Code by Dustin Boswell and Trevor Foucher.
- Clean Code by Robert C. Martin.